java.awt.geom
Class Area
- Cloneable, Shape
The Area class represents any area for the purpose of
Constructive Area Geometry (CAG) manipulations. CAG manipulations
work as an area-wise form of boolean logic, where the basic operations are:
Add (in boolean algebra: A or B)
Subtract (in boolean algebra: A and (not B) )
Intersect (in boolean algebra: A and B)
Exclusive Or
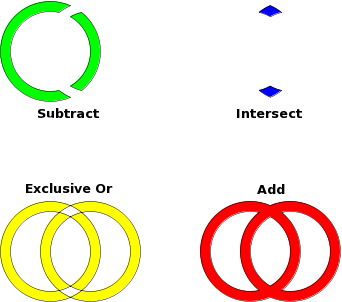
Above is an illustration of the CAG operations on two ring shapes.
The contains and intersects() methods are also more accurate than the
specification of #Shape requires.
Please note that constructing an Area can be slow
(Self-intersection resolving is proportional to the square of
the number of segments).
Area() - Constructs an empty Area
|
Area(Shape s) - Constructs an Area from any given Shape.
|
void | add(Area area) - Performs an add (union) operation on this area with another Area.
|
Object | clone() - Create a new area of the same run-time type with the same contents as
this one.
|
boolean | contains(double x, double y) - Determines if the point (x,y) is contained within this Area.
|
boolean | contains(double x, double y, double w, double h) - Determines if the rectangle specified by (x,y) as the upper-left
and with width w and height h is completely contained within this Area,
returns false otherwise.
This method should always produce the correct results, unlike for other
classes in geom.
|
boolean | contains(Point2D p) - Determines if the Point2D p is contained within this Area.
|
boolean | contains(Rectangle2D r) - Determines if the Rectangle2D specified by r is completely contained
within this Area, returns false otherwise.
This method should always produce the correct results, unlike for other
classes in geom.
|
Area | createTransformedArea(AffineTransform at) - Returns a new Area equal to this one, transformed
by the AffineTransform at.
|
boolean | equals(Area area) - Compares two Areas.
|
void | exclusiveOr(Area area) - Performs an exclusive-or operation on this Area.
|
Rectangle | getBounds() - Returns the bounds of this object in Rectangle format.
|
Rectangle2D | getBounds2D() - Returns the bounding box of the Area. Unlike the CubicCurve2D and
QuadraticCurve2D classes, this method will return the tightest possible
bounding box, evaluating the extreme points of each curved segment.
|
PathIterator | getPathIterator(AffineTransform at) - Returns a PathIterator object defining the contour of this Area,
transformed by at.
|
PathIterator | getPathIterator(AffineTransform at, double flatness) - Returns a flattened PathIterator object defining the contour of this
Area, transformed by at and with a defined flatness.
|
void | intersect(Area area) - Performs an intersection operation on this Area.
|
boolean | intersects(double x, double y, double w, double h) - Determines if the rectangle specified by (x,y) as the upper-left
and with width w and height h intersects any part of this Area.
|
boolean | intersects(Rectangle2D r) - Determines if the Rectangle2D specified by r intersects any
part of this Area.
|
boolean | isEmpty() - Returns whether this area encloses any area.
|
boolean | isPolygonal() - Determines whether the Area consists entirely of line segments
|
boolean | isRectangular() - Determines if the Area is rectangular.
This is strictly qualified.
|
boolean | isSingular() - Returns whether the Area consists of more than one simple
(non self-intersecting) subpath.
|
void | reset() - Clears the Area object, creating an empty area.
|
void | subtract(Area area) - Performs a subtraction operation on this Area.
|
void | transform(AffineTransform at) - Transforms this area by the AffineTransform at.
|
clone , equals , extends Object> getClass , finalize , hashCode , notify , notifyAll , toString , wait , wait , wait |
Area
public Area()
Constructs an empty Area
Area
public Area(Shape s)
Constructs an Area from any given Shape.
If the Shape is self-intersecting, the created Area will consist
of non-self-intersecting subpaths, and any inner paths which
are found redundant in accordance with the Shape's winding rule
will not be included.
s
- the shape (null
not permitted).
add
public void add(Area area)
Performs an add (union) operation on this area with another Area.
area
- - the area to be unioned with this one
clone
public Object clone()
Create a new area of the same run-time type with the same contents as
this one.
- clone in interface Object
contains
public boolean contains(double x,
double y)
Determines if the point (x,y) is contained within this Area.
- contains in interface Shape
x
- the x-coordinate of the point.y
- the y-coordinate of the point.
- true if the point is contained, false otherwise.
contains
public boolean contains(double x,
double y,
double w,
double h)
Determines if the rectangle specified by (x,y) as the upper-left
and with width w and height h is completely contained within this Area,
returns false otherwise.
This method should always produce the correct results, unlike for other
classes in geom.
- contains in interface Shape
x
- the x-coordinate of the rectangle.y
- the y-coordinate of the rectangle.w
- the width of the the rectangle.h
- the height of the rectangle.
true
if the rectangle is considered contained
contains
public boolean contains(Point2D p)
Determines if the Point2D p is contained within this Area.
- contains in interface Shape
true
if the point is contained, false
otherwise.
contains
public boolean contains(Rectangle2D r)
Determines if the Rectangle2D specified by r is completely contained
within this Area, returns false otherwise.
This method should always produce the correct results, unlike for other
classes in geom.
- contains in interface Shape
true
if the rectangle is considered contained
equals
public boolean equals(Area area)
Compares two Areas.
area
- the area to compare against this area (null
permitted).
true
if the areas are equal, and false
otherwise.
exclusiveOr
public void exclusiveOr(Area area)
Performs an exclusive-or operation on this Area.
area
- - the area to be XORed with this area.
getBounds
public Rectangle getBounds()
Returns the bounds of this object in Rectangle format.
Please note that this may lead to loss of precision.
- getBounds in interface Shape
getBounds2D
public Rectangle2D getBounds2D()
Returns the bounding box of the Area.
Unlike the CubicCurve2D and
QuadraticCurve2D classes, this method will return the tightest possible
bounding box, evaluating the extreme points of each curved segment.
- getBounds2D in interface Shape
intersect
public void intersect(Area area)
Performs an intersection operation on this Area.
area
- - the area to be intersected with this area.
intersects
public boolean intersects(double x,
double y,
double w,
double h)
Determines if the rectangle specified by (x,y) as the upper-left
and with width w and height h intersects any part of this Area.
- intersects in interface Shape
x
- the x-coordinate for the rectangle.y
- the y-coordinate for the rectangle.w
- the width of the rectangle.h
- the height of the rectangle.
true
if the rectangle intersects the area,
false
otherwise.
intersects
public boolean intersects(Rectangle2D r)
Determines if the Rectangle2D specified by r intersects any
part of this Area.
- intersects in interface Shape
r
- the rectangle to test intersection with (null
not permitted).
true
if the rectangle intersects the area,
false
otherwise.
isEmpty
public boolean isEmpty()
Returns whether this area encloses any area.
- true if the object encloses any area.
isPolygonal
public boolean isPolygonal()
Determines whether the Area consists entirely of line segments
- true if the Area lines-only, false otherwise
isRectangular
public boolean isRectangular()
Determines if the Area is rectangular.
This is strictly qualified. An area is considered rectangular if:
It consists of a single polygonal path.
It is oriented parallel/perpendicular to the xy axis
It must be exactly rectangular, i.e. small errors induced by
transformations may cause a false result, although the area is
visibly rectangular.
- true if the above criteria are met, false otherwise
isSingular
public boolean isSingular()
Returns whether the Area consists of more than one simple
(non self-intersecting) subpath.
- true if the Area consists of none or one simple subpath,
false otherwise.
reset
public void reset()
Clears the Area object, creating an empty area.
subtract
public void subtract(Area area)
Performs a subtraction operation on this Area.
area
- the area to be subtracted from this area.
Area.java -- represents a shape built by constructive area geometry
Copyright (C) 2002, 2004 Free Software Foundation
This file is part of GNU Classpath.
GNU Classpath is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; either version 2, or (at your option)
any later version.
GNU Classpath is distributed in the hope that it will be useful, but
WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
General Public License for more details.
You should have received a copy of the GNU General Public License
along with GNU Classpath; see the file COPYING. If not, write to the
Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
02110-1301 USA.
Linking this library statically or dynamically with other modules is
making a combined work based on this library. Thus, the terms and
conditions of the GNU General Public License cover the whole
combination.
As a special exception, the copyright holders of this library give you
permission to link this library with independent modules to produce an
executable, regardless of the license terms of these independent
modules, and to copy and distribute the resulting executable under
terms of your choice, provided that you also meet, for each linked
independent module, the terms and conditions of the license of that
module. An independent module is a module which is not derived from
or based on this library. If you modify this library, you may extend
this exception to your version of the library, but you are not
obligated to do so. If you do not wish to do so, delete this
exception statement from your version.