java.awt.geom
Class Ellipse2D
- Cloneable, Shape
Ellipse2D is the shape of an ellipse.
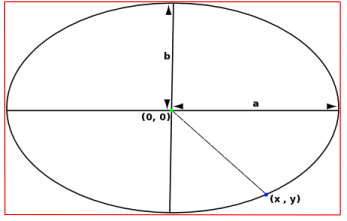
The ellipse is defined by it's bounding box (shown in red),
and is defined by the implicit curve:
(x/a)2 +
(y/b)2 = 1
Ellipse2D() - Ellipse2D is defined as abstract.
|
boolean | contains(double x, double y) - Determines if a point is contained within the ellipse.
|
boolean | contains(double x, double y, double w, double h) - Determines if a rectangle is completely contained within the
ellipse.
|
PathIterator | getPathIterator(AffineTransform at) - Returns a PathIterator object corresponding to the ellipse.
Note: An ellipse cannot be represented exactly in PathIterator
segments, the outline is thefore approximated with cubic
Bezier segments.
|
boolean | intersects(double x, double y, double w, double h) - Determines if a rectangle intersects any part of the ellipse.
|
clone , contains , contains , getBounds , getCenterX , getCenterY , getFrame , getHeight , getMaxX , getMaxY , getMinX , getMinY , getPathIterator , getWidth , getX , getY , intersects , isEmpty , setFrame , setFrame , setFrame , setFrameFromCenter , setFrameFromCenter , setFrameFromDiagonal , setFrameFromDiagonal |
clone , equals , extends Object> getClass , finalize , hashCode , notify , notifyAll , toString , wait , wait , wait |
Ellipse2D
protected Ellipse2D()
Ellipse2D is defined as abstract.
Implementing classes are Ellipse2D.Float and Ellipse2D.Double.
contains
public boolean contains(double x,
double y)
Determines if a point is contained within the ellipse.
- contains in interface Shape
x
- - x coordinate of the point.y
- - y coordinate of the point.
- true if the point is within the ellipse, false otherwise.
contains
public boolean contains(double x,
double y,
double w,
double h)
Determines if a rectangle is completely contained within the
ellipse.
- contains in interface Shape
x
- - x coordinate of the upper-left corner of the rectangley
- - y coordinate of the upper-left corner of the rectanglew
- - width of the rectangleh
- - height of the rectangle
- true if the rectangle is completely contained, false otherwise.
getPathIterator
public PathIterator getPathIterator(AffineTransform at)
Returns a PathIterator object corresponding to the ellipse.
Note: An ellipse cannot be represented exactly in PathIterator
segments, the outline is thefore approximated with cubic
Bezier segments.
- getPathIterator in interface Shape
at
- an optional transform.
intersects
public boolean intersects(double x,
double y,
double w,
double h)
Determines if a rectangle intersects any part of the ellipse.
- intersects in interface Shape
x
- - x coordinate of the upper-left corner of the rectangley
- - y coordinate of the upper-left corner of the rectanglew
- - width of the rectangleh
- - height of the rectangle
- true if the rectangle intersects the ellipse, false otherwise.
Ellipse2D.java -- represents an ellipse in 2-D space
Copyright (C) 2000, 2002, 2004 Free Software Foundation
This file is part of GNU Classpath.
GNU Classpath is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; either version 2, or (at your option)
any later version.
GNU Classpath is distributed in the hope that it will be useful, but
WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
General Public License for more details.
You should have received a copy of the GNU General Public License
along with GNU Classpath; see the file COPYING. If not, write to the
Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
02110-1301 USA.
Linking this library statically or dynamically with other modules is
making a combined work based on this library. Thus, the terms and
conditions of the GNU General Public License cover the whole
combination.
As a special exception, the copyright holders of this library give you
permission to link this library with independent modules to produce an
executable, regardless of the license terms of these independent
modules, and to copy and distribute the resulting executable under
terms of your choice, provided that you also meet, for each linked
independent module, the terms and conditions of the license of that
module. An independent module is a module which is not derived from
or based on this library. If you modify this library, you may extend
this exception to your version of the library, but you are not
obligated to do so. If you do not wish to do so, delete this
exception statement from your version.